Robbie Hatley's Solutions, in Perl, for The Weekly Challenge #297 ("Contiguous Sub-Arrays" and "Semi-Ordered Permutations")
For those not familiar with "The Weekly Challenge", it is a weekly programming puzzle with two parts, cycling every Sunday. You can find it here:
The Weekly Challenge for the week of 2024-11-24 through 2024-11-30 is #297.
The tasks for challenge #297 are as follows:
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Task 297-1: Contiguous Sub-Arrays Submitted by: Mohammad Sajid Anwar Write a script which, given an array of 1-digit-binary integers (0s and 1s), determines the maximum length of contiguous sub-arrays with equal numbers of 0s and 1s. Example #1: Input: @binary = (1, 0) Output: 2 (1, 0) is the longest contiguous subarray with an equal number of 0 and 1. Example #2: Input: @binary = (0, 1, 0) Output: 2 (1, 0) or (0, 1) is the longest contiguous subarray with an equal number of 0 and 1. Example #3: Input: @binary = (0, 0, 0, 0, 0) Output: 0 Example #4: Input: @binary = (0, 1, 0, 0, 1, 0) Output: 4 (1,0,0,1)
I'll take the approach of summing the elements of each sub-array by feeding array slices to the "sum0" function from CPAN module "List::Util", then dividing the length of each sub-array by its sum; a sub-array will have equal numbers of 0s and 1s if-and-only-if length == 2*sum. (It's tempting to test for length/sum == 2, but that would be a disaster because sum will sometimes be 0, causing divide-by-zero errors.) Helping will be the fact that only even-length sub-arrays need be checked, which cuts the work in half.
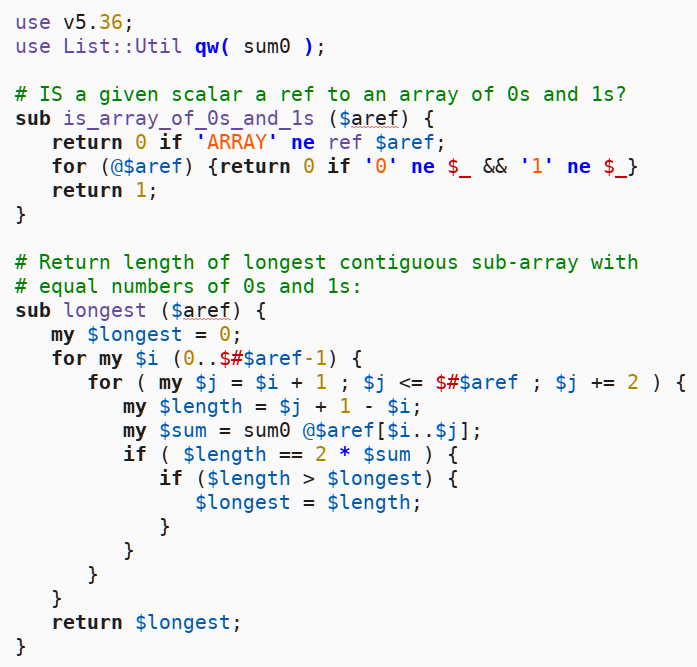
Robbie Hatley's Perl Solution to The Weekly Challenge 297-1
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Task 297-2: Semi-Ordered Permutation Submitted by: Mohammad Sajid Anwar Write a script which determines the minimum number of swaps of adjacent elements necessary to make a given permutation of the first n positive integers (for some positive integer n) "semi-ordered" (meaning that the first element is 1 and the last element is n). Example #1: Input: @ints = (2, 1, 4, 3) Output: 2 Swap 2 <=> 1 => (1, 2, 4, 3) Swap 4 <=> 3 => (1, 2, 3, 4) Example #2: Input: @ints = (2, 4, 1, 3) Output: 3 Swap 4 <=> 1 => (2, 1, 4, 3) Swap 2 <=> 1 => (1, 2, 4, 3) Swap 4 <=> 3 => (1, 2, 3, 4) Example #3: Input: @ints = (1, 3, 2, 4, 5) Output: 0 (Already a semi-ordered permutation.)
This task is much easier than the first, because it's just a matter of counting swaps needed. And we don't even have to do any real swaps! Since we're only interested in the "1" and "n" elements, we need only count the number of swaps that would have been needed to pull "1" to the beginning and "n" to the end (keeping in-mind that if "1" and "n" cross-over, we save 1 swap, so we need to reduce our swaps count by 1 in that case).
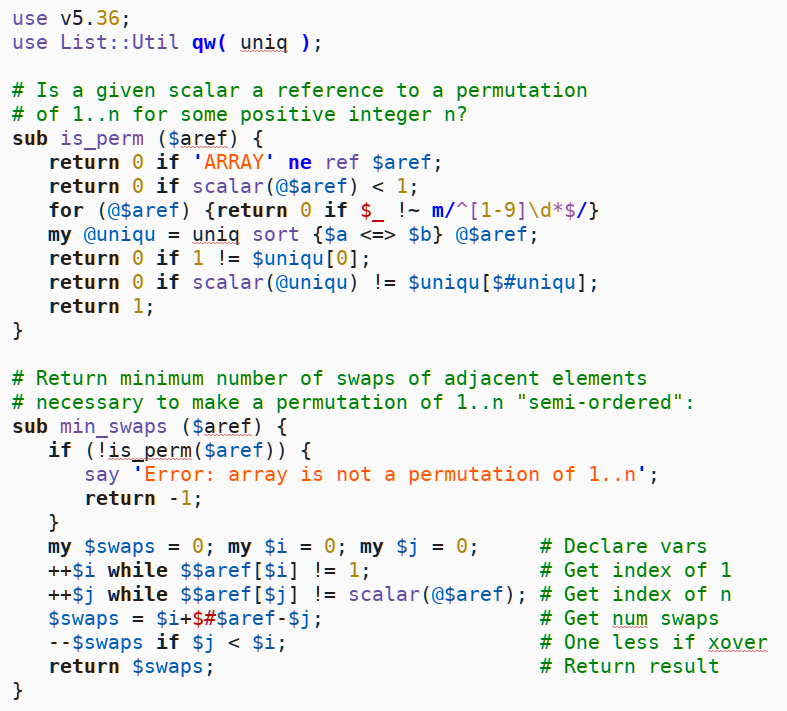
Robbie Hatley's Perl Solution to The Weekly Challenge 297-2
That's it for challenge 297; see you on challenge 298!
Comments
Post a Comment