Robbie Hatley's Solutions, in Perl, for The Weekly Challenge #299 (Word Replacements and Searches)
For those not familiar with "The Weekly Challenge", it is a weekly programming puzzle with two parts, cycling every Sunday. You can find it here:
The Weekly Challenge for the week of 2024-12-08 through 2024-12-14 is #299.
The tasks for challenge #299 are as follows:
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Task 299-1: Replace Words Submitted by: Mohammad Sajid Anwar You are given an array of words and a sentence. Write a script to replace all words in the given sentence that start with any of the words in the given array. Example #1: Input: @words = ("cat", "bat", "rat") $sentence = "the cattle was rattle by the battery" Output: "the cat was rat by the bat" Example #2: Input: @words = ("a", "b", "c") $sentence = "aab aac and cac bab" Output: "a a a c b" Example #3: Input: @words = ("man", "bike") $sentence = "the manager was hit by a biker" Output: "the man was hit by a bike"
My approach was to first split the sentence into a list of words, then for each word/replacement pair, if the word starts with the replacement, then change every instance of that word within the sentence to that replacement word.
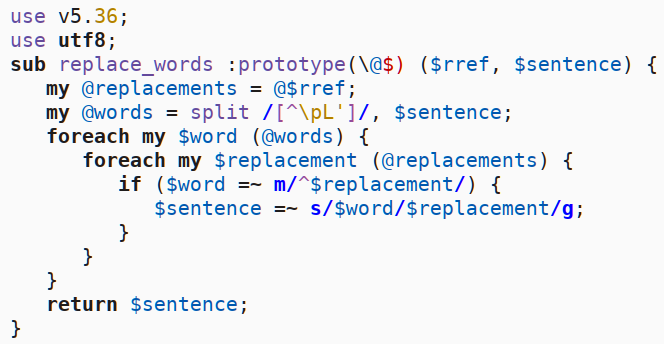
Robbie Hatley's Perl Solution to The Weekly Challenge 299-1
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Task 299-2: Word Search Submitted by: Mohammad Sajid Anwar You are given a grid of characters and a string. Write a script to determine whether the given string can be found in the given grid of characters. You may start anywhere and take any orthogonal path, but may not reuse a grid cell. Example #1: Input: @chars = ['A', 'B', 'D', 'E'], ['C', 'B', 'C', 'A'], ['B', 'A', 'A', 'D'], ['D', 'B', 'B', 'C'], $str = 'BDCA' Output: true Example #2: Input: @chars = ['A', 'A', 'B', 'B'], ['C', 'C', 'B', 'A'], ['C', 'A', 'A', 'A'], ['B', 'B', 'B', 'B'], $str = 'ABAC' Output: false Example #3: Input: @chars = ['B', 'A', 'B', 'A'], ['C', 'C', 'C', 'C'], ['A', 'B', 'A', 'B'], ['B', 'B', 'A', 'A'], $str = 'CCCAA' Output: true
I made a subroutine which uses this approach: From each possible starting point, try building paths from that point which obey these three restrictions:
- Path doesn't go out-of-bounds
- Path doesn't re-use any cells
- Path so-far matches relevant characters of string
If any path is found which fully matches string, return 1; else return 0.
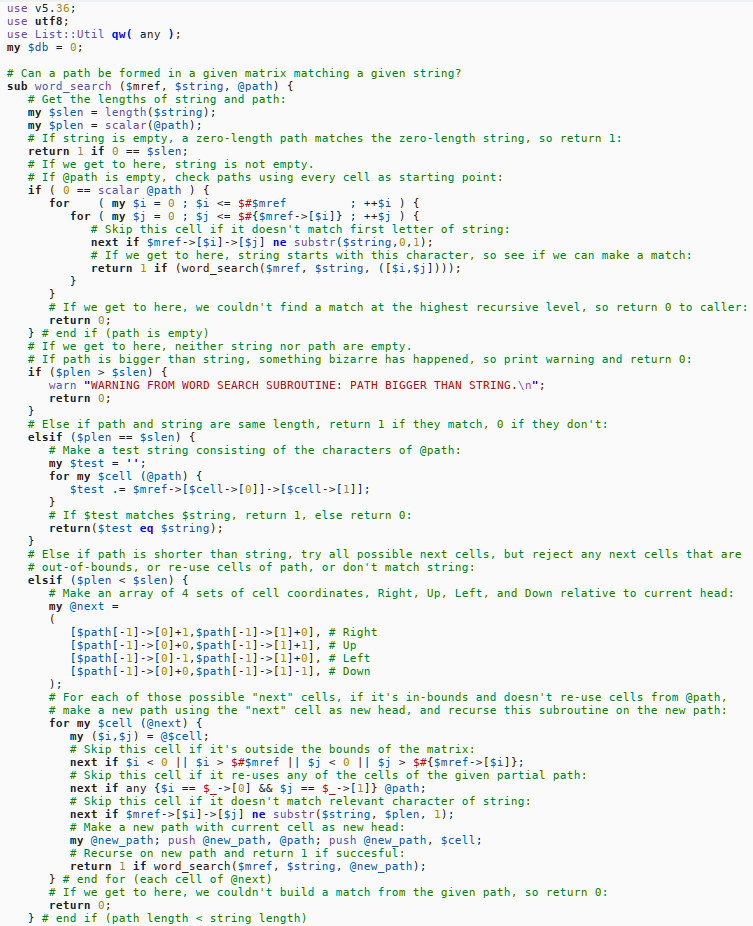
Robbie Hatley's Perl Solution to The Weekly Challenge 299-2
That's it for challenge 299; see you on challenge 300!
Comments
Post a Comment