Robbie Hatley's Solutions, in Perl, for The Weekly Challenge #301 ("Greatest Number" and "Hamming Distance")
For those not familiar with "The Weekly Challenge", it is a weekly programming puzzle with two parts, cycling every Sunday. You can find it here:
The Weekly Challenge for the week of 2024-12-23 through 2024-12-29 is #301.
The tasks for challenge #301 are as follows:
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Task 301-1: Largest Number Submitted by: Mohammad Sajid Anwar You are given a list of positive integers, @ints. Write a script to arrange all the elements in the given list such that they form the largest number and return it. Example #1: Input: @ints = (20, 3) Output: 320 Example #2: Input: @ints = (3, 30, 34, 5, 9) Output: 9534330
While it's tempting to just "sort and join", while that would work with some numbers (eg, Example #1), it wouldn't work with others (eg, Example #2). So I'll use the non-OOP "permute" function from my favorite CPAN module, "Math::Combinatorics", to get all permutations, join each, and see which is greatest. (I'm sure there are ways of doing this with smaller big-O, but I've got other things to do this week, so I'll go for the Godzilla smash instead of the surgical precision.)
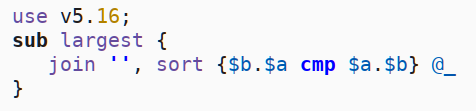
Robbie Hatley's Perl Solution to The Weekly Challenge 301-1
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Task 301-2: Hamming Distance Submitted by: Mohammad Sajid Anwar You are given an array of integers, @ints. Write a script to return the sum of Hamming distances between all the pairs of the integers in the given array of integers. The Hamming distance between two integers is the number of places in which their binary representations differ. Example #1: Input: @ints = (4, 14, 2) Output: 6 Binary representation: 4 => 0100 14 => 1110 2 => 0010 HammingDistance( 4, 14) + HammingDistance( 4, 2) + HammingDistance(14, 2) = 2 + 2 + 2 = 6. Example #2: Input: @ints = (4, 14, 4) Output: 4
Firstly, I'll assume that "integers" means "non-negative integers", as negative integers have no predetmined binary representation, as that's dependent on the encoding and bit-width used by one's CPU and programming language.
That being stipulated, I'll use two subs: one called "H" to get the Hamming distance, and one called "H_sum" to get the summation of the Hamming distances.
My "H" sub will use the ridiculously-simple approach of bitwise-xor-ing-together the two numbers, which will yield a binary number having exactly as many 1s in it as the Hamming Distance between the two numbers. Then use sprintf("%b", $x^$y) to get a string copy of the binary representation. Then use a "s/0//gr" operator to get rid of all of the 0s leaving only the 1s. Then use "length" to count the 1s.
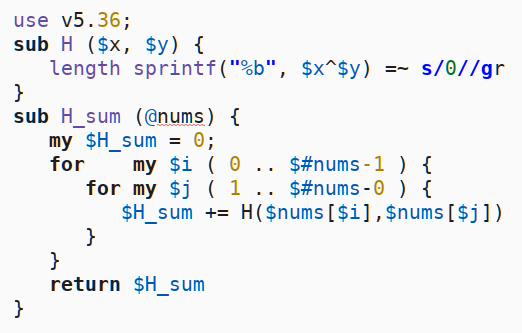
Robbie Hatley's Perl Solution to The Weekly Challenge 301-2
That's it for challenge 301; see you on challenge 302!
Comments
Post a Comment