Robbie Hatley's Solutions, in Perl, for The Weekly Challenge #306 ("Odd Sum" and "Last Element")
For those not familiar with "The Weekly Challenge", it is a weekly programming puzzle with two parts, cycling every Sunday. You can find it here:
The Weekly Challenge for the week of 2025-01-27 through 2025-02-02 is #306.
The tasks for challenge #306 are as follows:
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Task 306-1: "Odd Sum" Submitted by: Mohammad Sajid Anwar You are given an array of positive integers, @ints. Write a script to return the sum of all possible odd-length subarrays of the given array. A subarray is a contiguous subsequence of the array. Example #1: Input: @ints = (2, 5, 3, 6, 4) Output: 77 Odd length sub-arrays: (2) => 2 (5) => 5 (3) => 3 (6) => 6 (4) => 4 (2, 5, 3) => 10 (5, 3, 6) => 14 (3, 6, 4) => 13 (2, 5, 3, 6, 4) => 20 Sum => 2 + 5 + 3 + 6 + 4 + 10 + 14 + 13 + 20 => 77 Example #2: Input: @ints = (1, 3) Output: 4
I'll solve this problem by using nested for loops to generate all valid odd-length blocks of contiguous indices for the given array, sum each with sum0 (from CPAN module "List::Util"), add each such sum to a variable "$sum", then return $sum.
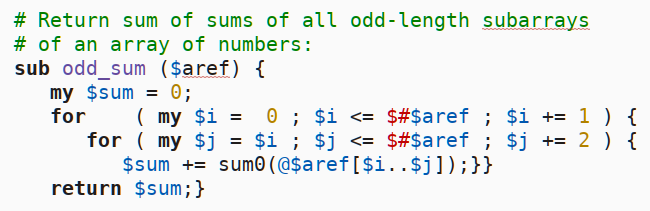
Robbie Hatley's Perl Solution to The Weekly Challenge 306-1
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ Task 306-2: "Last Element" Submitted by: Mohammad Sajid Anwar You are given a array of integers, @ints. Write a script to play a game where you pick two biggest integers in the given array, say x and y. Then do the following: a) if x == y then remove both from the given array b) if x != y then remove x and replace y with (y - x) At the end of the game, there is at most one element left. Return the last element if found otherwise return 0. Example #1: Input: @ints = (3, 8, 5, 2, 9, 2) Output: 1 Step 1: pick 8 and 9 => (3, 5, 2, 1, 2) Step 2: pick 3 and 5 => (2, 2, 1, 2) Step 3: pick 2 and 1 => (1, 2, 2) Step 4: pick 2 and 1 => (1, 2) Step 5: pick 1 and 2 => (1) Example #2: Input: @ints = (3, 2, 5) Output: 0 Step 1: pick 3 and 5 => (2, 2) Step 2: pick 2 and 2 => ()
Since we're going to end up boiling each incoming array down to 1 or 0 elements, order doesn't matter, so I'll start by doing reverse numeric sort on the array. And each time an element is replaced, I'll sort the array again. That way, "the two biggest integers" will always be the first two elements. I'll then keep deleting and replacing as specified in the problem description, except that if the two largest integers are equal, I'll replace them with 0. That way, I'll always end up with exactly 1 integer left in the array (which may be zero or non-zero), and I'll present that integer as the output.
By the way, I note that Example #1 doesn't obey the problem description's mandate "pick two biggest integers", as Steps 4 and 5 both pick "2 and 1" when in either case the two biggest integers would have been "2 and 2". The problem description doesn't specify "two biggest UNIQUE integers", so I elect to consider steps 4 and 5 of Example #1 to be "in-error" rather than re-write the problem description.
I also note that by following the problem description as-written (ie, "the two biggest integers present, whether unique or identical"), in Example 1 the answer 1 is also obtained, though in a very different way.
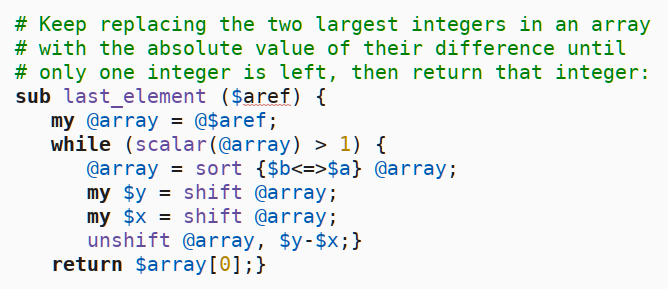
Robbie Hatley's Perl Solution to The Weekly Challenge 306-2
That's it for challenge 306; see you on challenge 307!
Comments
Post a Comment